here's a method that calculates the number of days between 2 dates:
...
public static long getDateDiffInDays(Date date1, Date date2) {
long diffInMillis = date2.getTime() - date1.getTime();
return TimeUnit.MILLISECONDS.toDays(diffInMillis);
}
...
and here's some test code you can use:
...
public static void main(String[] args) {
// today
Calendar today = Calendar.getInstance();
// tomorrow
Calendar tomorrow = Calendar.getInstance();
tomorrow.add(Calendar.DAY_OF_YEAR, 1);
System.out.println(getDateDiffInDays(today.getTime(), tomorrow.getTime()));
}
...
OUTPUT:
1
In Calculating the Difference Between Two Java Date Instances there's a great, general method for getting differences between dates:
public static long getDateDiff(Date date1, Date date2, TimeUnit timeUnit) {
long diffInMillies = date2.getTime() - date1.getTime();
return timeUnit.convert(diffInMillies,TimeUnit.MILLISECONDS);
}
and here's some test code:
public static void main(String[] args) {
Calendar today = Calendar.getInstance();
Calendar tomorrow = Calendar.getInstance();
tomorrow.add(Calendar.DAY_OF_YEAR, 1);
// Calculate the difference in days. OUTPUT: 1
System.out.println(getDateDiff(today.getTime(), tomorrow.getTime(), TimeUnit.DAYS));
// Calculate the difference in hours. OUTPUT: 24
System.out.println(getDateDiff(today.getTime(), tomorrow.getTime(), TimeUnit.HOURS));
// Now add 6 hours to "tomorrow"'s point in time
tomorrow.add(Calendar.HOUR_OF_DAY, 6);
// Calculate the difference in hours. OUTPUT: 30
System.out.println(getDateDiff(today.getTime(), tomorrow.getTime(), TimeUnit.HOURS));
}
Here's a test class you can play around with: http://pastebin.com/PteRYg2d
Thanks also to:
milliseconds to days
Relevant Java API documentation:
http://docs.oracle.com/javase/6/docs/api/java/util/concurrent/TimeUnit.html
http://docs.oracle.com/javase/6/docs/api/java/util/Calendar.html
http://docs.oracle.com/javase/6/docs/api/java/util/Date.html
IT, computer and programming tutorials and tips that i couldnt find anywhere else using google, from my daily work as a Senior Developer of solutions using Java and Linux.
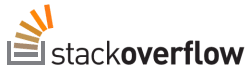
The best place to *find* answers to programming/development questions, imo, however it's the *worst* place to *ask* questions (if your first question/comment doesn't get any up-rating/response, then u can't ask anymore questions--ridiculously unrealistic), but again, a great reference for *finding* answers.
My Music (Nickleus)
Subscribe to:
Post Comments (Atom)
No comments:
Post a Comment