especially in large java programs, it's important to explicitly set unused (or no longer used) variables/objects/references to null to make them available for garbage collection.
Here is an example of "nulling the reference":
public class GarbageCollectionExample {
public static void main(String[] args) {
StringBuffer x = new StringBuffer("test");
System.out.println(x);
// the StringBuffer "test" is not available for GC yet
x = null;
// the StringBuffer "test" is now available for GC
}
}
Here is an example of "reassigning a reference value":
public class GarbageCollectionExample {
public static void main(String[] args) {
StringBuffer x = new StringBuffer("test");
StringBuffer y = new StringBuffer("asdf");
System.out.println(x);
// the StringBuffer "test" is not available for GC yet
x = y;
// the StringBuffer "test" is now available for GC}
the StringBuffer "test" is no longer reachable because "x" now references the StringBuffer "asdf".
(taken from pages 257-258 in "Sun Certified Programmer for Java 6 Study Guide")
IT, computer and programming tutorials and tips that i couldnt find anywhere else using google, from my daily work as a Senior Developer of solutions using Java and Linux.
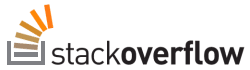
The best place to *find* answers to programming/development questions, imo, however it's the *worst* place to *ask* questions (if your first question/comment doesn't get any up-rating/response, then u can't ask anymore questions--ridiculously unrealistic), but again, a great reference for *finding* answers.
My Music (Nickleus)
20130715
java tip of the day - explicitly set unused (or no longer used) variables/objects/references to null to make them available for garbage collection
Subscribe to:
Post Comments (Atom)
No comments:
Post a Comment