I wanted to find out if an ArrayList of String arrays (String[]) was mutable or not and i found out that it is. Here's some test code to play with:
package com.test;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
public class ArrayMutationTest {
public static void main(String[] args) {
List<String[]> objList = new ArrayList<String[]>();
String[] l1 = {"a",""}; // (K)ey, (V)alue
String[] l2 = {"b",""};
objList.add(l1);
objList.add(l2);
String csStringK = "a,b"; // (K)eys
String csStringV = "1,2"; // (V)alues
List<String> filterFieldKeys = Arrays.asList(csStringK.split("\\s*,\\s*"));
List<String> filterFieldValues = Arrays.asList(csStringV.split("\\s*,\\s*"));
for(Object[] ff : objList) {
String filterKey = (String) ff[0];
int i = filterFieldKeys.indexOf(filterKey);
if(i != -1) {
ff[1] = filterFieldValues.get(i);
}
}
}
}
--------
after populating objList first, it looks like this:
["a", ""]
["b", ""]
after setting values in the for-loop it looks like this:
["a", "1"]
["b", "2"]
IT, computer and programming tutorials and tips that i couldnt find anywhere else using google, from my daily work as a Senior Developer of solutions using Java and Linux.
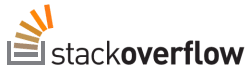
The best place to *find* answers to programming/development questions, imo, however it's the *worst* place to *ask* questions (if your first question/comment doesn't get any up-rating/response, then u can't ask anymore questions--ridiculously unrealistic), but again, a great reference for *finding* answers.
My Music (Nickleus)
Subscribe to:
Post Comments (Atom)
No comments:
Post a Comment