if you have duplicate lines in a text file, in this format:
line 1
line 1
line 2
line 2
...
...
this regexp code will remove the duplicate lines:
search: (.*)\n\1
replace: \1\n
regular expression syntax for geany
IT, computer and programming tutorials and tips that i couldnt find anywhere else using google, from my daily work as a Senior Developer of solutions using Java and Linux.
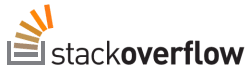
The best place to *find* answers to programming/development questions, imo, however it's the *worst* place to *ask* questions (if your first question/comment doesn't get any up-rating/response, then u can't ask anymore questions--ridiculously unrealistic), but again, a great reference for *finding* answers.
My Music (Nickleus)
20130629
20130625
uploading sf2 soundfont files to archive.org doesn't show the files on the item page
i uploaded a bunch of SF2 soundfonts to archive.org:
Nickleus SF2 SoundFont Collection
but after the upload was finished, none of the files i had uploaded were shown on the main item page.
here's the archive support thread: https://archive.org/post/934181/sf2-format-doesnt-list-files-on-details-page-change-identifier
jeff kaplan found out that the mediatype needed to be set to software, because archive.org doesn't automatically recognize the SF2 file type for some reason.
Nickleus SF2 SoundFont Collection
but after the upload was finished, none of the files i had uploaded were shown on the main item page.
here's the archive support thread: https://archive.org/post/934181/sf2-format-doesnt-list-files-on-details-page-change-identifier
jeff kaplan found out that the mediatype needed to be set to software, because archive.org doesn't automatically recognize the SF2 file type for some reason.
20130613
java - are "ArrayList"s of String arrays (String[]) mutable? yes
I wanted to find out if an ArrayList of String arrays (String[]) was mutable or not and i found out that it is. Here's some test code to play with:
package com.test;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
public class ArrayMutationTest {
public static void main(String[] args) {
List<String[]> objList = new ArrayList<String[]>();
String[] l1 = {"a",""}; // (K)ey, (V)alue
String[] l2 = {"b",""};
objList.add(l1);
objList.add(l2);
String csStringK = "a,b"; // (K)eys
String csStringV = "1,2"; // (V)alues
List<String> filterFieldKeys = Arrays.asList(csStringK.split("\\s*,\\s*"));
List<String> filterFieldValues = Arrays.asList(csStringV.split("\\s*,\\s*"));
for(Object[] ff : objList) {
String filterKey = (String) ff[0];
int i = filterFieldKeys.indexOf(filterKey);
if(i != -1) {
ff[1] = filterFieldValues.get(i);
}
}
}
}
--------
after populating objList first, it looks like this:
["a", ""]
["b", ""]
after setting values in the for-loop it looks like this:
["a", "1"]
["b", "2"]
package com.test;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
public class ArrayMutationTest {
public static void main(String[] args) {
List<String[]> objList = new ArrayList<String[]>();
String[] l1 = {"a",""}; // (K)ey, (V)alue
String[] l2 = {"b",""};
objList.add(l1);
objList.add(l2);
String csStringK = "a,b"; // (K)eys
String csStringV = "1,2"; // (V)alues
List<String> filterFieldKeys = Arrays.asList(csStringK.split("\\s*,\\s*"));
List<String> filterFieldValues = Arrays.asList(csStringV.split("\\s*,\\s*"));
for(Object[] ff : objList) {
String filterKey = (String) ff[0];
int i = filterFieldKeys.indexOf(filterKey);
if(i != -1) {
ff[1] = filterFieldValues.get(i);
}
}
}
}
--------
after populating objList first, it looks like this:
["a", ""]
["b", ""]
after setting values in the for-loop it looks like this:
["a", "1"]
["b", "2"]
20130607
sony xperia ubuntu 13.04 usb not connected can't read storage sd card, but the phone charges
i tried stopping, killing and clear cache for all processes related to usb and mtp, but still couldn't get ubuntu to recognize my mobile cell phone.
then i went here on my sony xperia:
settings > wireless and network settings > tethering (but didn't do/click anything)
then after about 30 seconds the phone connected to ubuntu.... weird.
sometimes i get the following error message in ubuntu:
Unable to access “SEMC HSUSB Device”
Unable to open MTP device '[usb:003,003]'
quite the headache...
UPDATE
it seems like maybe this has something to do with it:
http://paulphilippov.com/articles/how-to-fix-device-not-accepting-address-error
"most probably it’s a result of hardware failure rather than a driver or kernel bug. USB has an over-current protection, which gets triggered when power consumption from the port is too high.
Unplug all USB devices from PC, turn power off, and wait a minute or two. Plug everything back and boot into Linux."
then i went here on my sony xperia:
settings > wireless and network settings > tethering (but didn't do/click anything)
then after about 30 seconds the phone connected to ubuntu.... weird.
sometimes i get the following error message in ubuntu:
Unable to access “SEMC HSUSB Device”
Unable to open MTP device '[usb:003,003]'
quite the headache...
UPDATE
it seems like maybe this has something to do with it:
http://paulphilippov.com/articles/how-to-fix-device-not-accepting-address-error
"most probably it’s a result of hardware failure rather than a driver or kernel bug. USB has an over-current protection, which gets triggered when power consumption from the port is too high.
Unplug all USB devices from PC, turn power off, and wait a minute or two. Plug everything back and boot into Linux."
java - how to find the number of days difference between two java.util.Date objects using core java api and not Joda Time
here's a method that calculates the number of days between 2 dates:
...
public static long getDateDiffInDays(Date date1, Date date2) {
long diffInMillis = date2.getTime() - date1.getTime();
return TimeUnit.MILLISECONDS.toDays(diffInMillis);
}
...
and here's some test code you can use:
...
public static void main(String[] args) {
// today
Calendar today = Calendar.getInstance();
// tomorrow
Calendar tomorrow = Calendar.getInstance();
tomorrow.add(Calendar.DAY_OF_YEAR, 1);
System.out.println(getDateDiffInDays(today.getTime(), tomorrow.getTime()));
}
...
OUTPUT:
1
In Calculating the Difference Between Two Java Date Instances there's a great, general method for getting differences between dates:
public static long getDateDiff(Date date1, Date date2, TimeUnit timeUnit) {
long diffInMillies = date2.getTime() - date1.getTime();
return timeUnit.convert(diffInMillies,TimeUnit.MILLISECONDS);
}
and here's some test code:
public static void main(String[] args) {
Calendar today = Calendar.getInstance();
Calendar tomorrow = Calendar.getInstance();
tomorrow.add(Calendar.DAY_OF_YEAR, 1);
// Calculate the difference in days. OUTPUT: 1
System.out.println(getDateDiff(today.getTime(), tomorrow.getTime(), TimeUnit.DAYS));
// Calculate the difference in hours. OUTPUT: 24
System.out.println(getDateDiff(today.getTime(), tomorrow.getTime(), TimeUnit.HOURS));
// Now add 6 hours to "tomorrow"'s point in time
tomorrow.add(Calendar.HOUR_OF_DAY, 6);
// Calculate the difference in hours. OUTPUT: 30
System.out.println(getDateDiff(today.getTime(), tomorrow.getTime(), TimeUnit.HOURS));
}
Here's a test class you can play around with: http://pastebin.com/PteRYg2d
Thanks also to:
milliseconds to days
Relevant Java API documentation:
http://docs.oracle.com/javase/6/docs/api/java/util/concurrent/TimeUnit.html
http://docs.oracle.com/javase/6/docs/api/java/util/Calendar.html
http://docs.oracle.com/javase/6/docs/api/java/util/Date.html
...
public static long getDateDiffInDays(Date date1, Date date2) {
long diffInMillis = date2.getTime() - date1.getTime();
return TimeUnit.MILLISECONDS.toDays(diffInMillis);
}
...
and here's some test code you can use:
...
public static void main(String[] args) {
// today
Calendar today = Calendar.getInstance();
// tomorrow
Calendar tomorrow = Calendar.getInstance();
tomorrow.add(Calendar.DAY_OF_YEAR, 1);
System.out.println(getDateDiffInDays(today.getTime(), tomorrow.getTime()));
}
...
OUTPUT:
1
In Calculating the Difference Between Two Java Date Instances there's a great, general method for getting differences between dates:
public static long getDateDiff(Date date1, Date date2, TimeUnit timeUnit) {
long diffInMillies = date2.getTime() - date1.getTime();
return timeUnit.convert(diffInMillies,TimeUnit.MILLISECONDS);
}
and here's some test code:
public static void main(String[] args) {
Calendar today = Calendar.getInstance();
Calendar tomorrow = Calendar.getInstance();
tomorrow.add(Calendar.DAY_OF_YEAR, 1);
// Calculate the difference in days. OUTPUT: 1
System.out.println(getDateDiff(today.getTime(), tomorrow.getTime(), TimeUnit.DAYS));
// Calculate the difference in hours. OUTPUT: 24
System.out.println(getDateDiff(today.getTime(), tomorrow.getTime(), TimeUnit.HOURS));
// Now add 6 hours to "tomorrow"'s point in time
tomorrow.add(Calendar.HOUR_OF_DAY, 6);
// Calculate the difference in hours. OUTPUT: 30
System.out.println(getDateDiff(today.getTime(), tomorrow.getTime(), TimeUnit.HOURS));
}
Here's a test class you can play around with: http://pastebin.com/PteRYg2d
Thanks also to:
milliseconds to days
Relevant Java API documentation:
http://docs.oracle.com/javase/6/docs/api/java/util/concurrent/TimeUnit.html
http://docs.oracle.com/javase/6/docs/api/java/util/Calendar.html
http://docs.oracle.com/javase/6/docs/api/java/util/Date.html
20130605
20130604
[SOLVED] ejb3 jboss 7 postconstruct annotation not called/working
we had a weird problem where a @PostConstruct (javax.annotation.PostConstruct) annotation wasn't getting called in one of our ejbs.
we found out that the problem was some old ejb2 lifecycle methods needed to be removed from the java class:
public void ejbRemove() {}
public void ejbActivate() {}
public void ejbPassivate() {}
public void ejbCreate() {}
when we built the project again and restarted jboss, then @PostConstruct worked :)
we found out that the problem was some old ejb2 lifecycle methods needed to be removed from the java class:
public void ejbRemove() {}
public void ejbActivate() {}
public void ejbPassivate() {}
public void ejbCreate() {}
when we built the project again and restarted jboss, then @PostConstruct worked :)
Subscribe to:
Posts (Atom)